In JavaScript, you cannot escape doing a lot of work with arrays. In this article, we will take a deep dive into how you can work with arrays efficiently and with little code to get the desired result you want.
1. Array.map()
This function is useful if you want to perform an operation on all the data stored in your array and return a new array. For example:
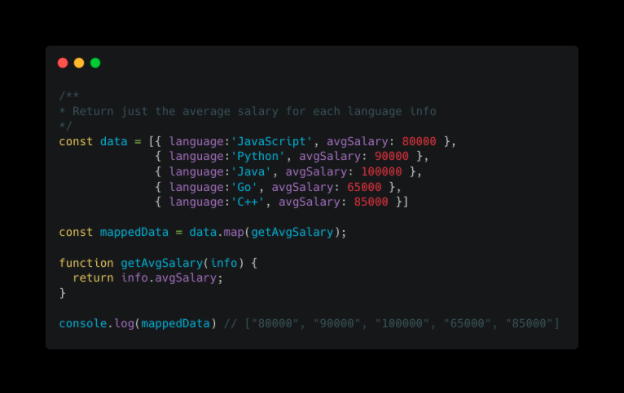
2. Array.filter()
What the function filter does is, it returns a new array based on the condition given in a function. For example, if we wanted to return all salaries greater than 80k what will that look like?
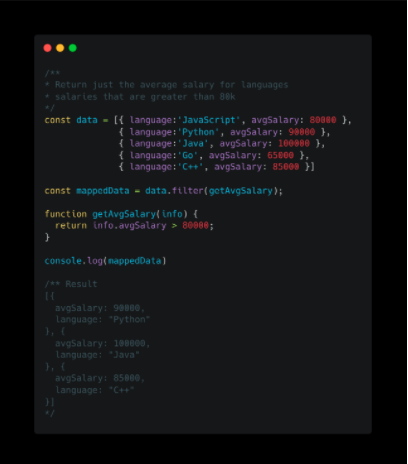
3. Array.reduce()
This function executes an array on each data in the array, in order, to pass the return value from the previous calculations to the next calculation. The final result is normally a single value. An initial value can be passed in to start, however, the default is 0.
This might be complex to understand, however, let’s simplify this with an example of summing elements in an array:
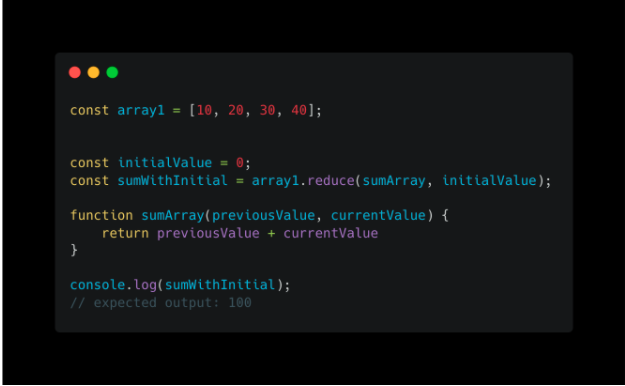
This function starts with an
- initial value of 0, at this point previous value is 0 and current value is 10
- So 0+10 = 10, 10 is now previousValue, then 10 + currentValue(20) = 30.
- 30 (as result from the previous computation) will now be the previous value.
- Then 30 + 30 = 60. Then 60 + 40 = 100
If no initial value is given then the first iteration is not run instead it starts computing from the index of 10 and 20 in the array. What happens when you change the initial value to be another number greater than 0?
We just looked at three popular Higher-Order Functions in JavaScript. A “higher-order function” is a function that accepts functions as parameters and/or returns a function. This is an important concept to know in JavaScript.
Other cool things you can do to manipulate JavaScript Arrays are:
4. Spread operator
The spread operator is used to unpack an array, The spread operator allows you to spread out elements of an iterable object such as an array. The annotation looks like this `…`. This can also be confused with the rest parameter, which collects all remaining arguments of a function into an array.
Example of Spread Operator
In this example it doesn’t also matter where the spread operator is, it can be at the front ([…odd, 2,4,6]), middle ([2,4, …odd, 6]) or end ([2,4,6 …odd]).
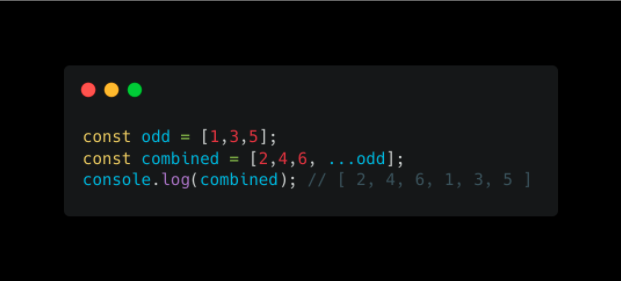
Example of Rest Parameter:
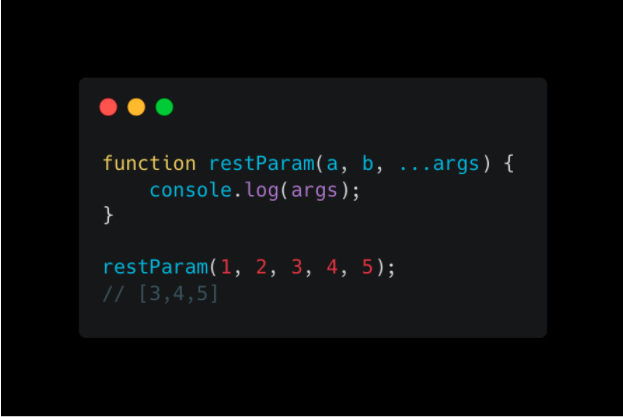
More ideas on what you can do with the Spread Operator
Combining two arrays
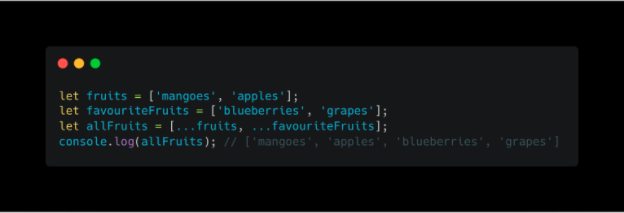
Copying an Array
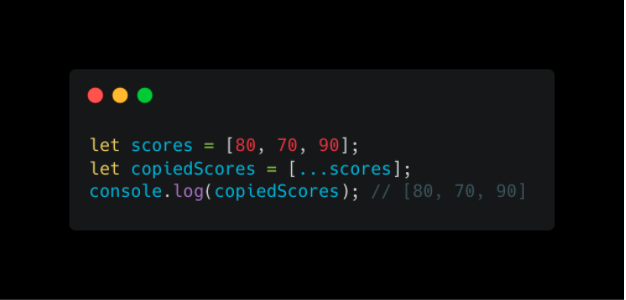
Moving Array Data From One to Another
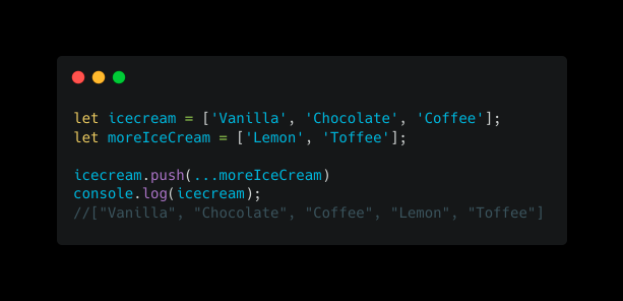
5. Array Destructuring
Array destructuring is another ES6 JavaScript functionality that allows values in an array to be accessed easily. Normally we try to access array values with their index numbers, but with destructuring, we can do this in a much cleaner way.
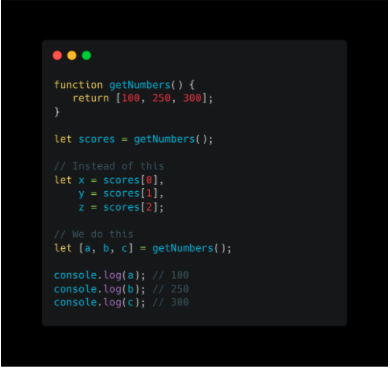
The variables a, b and c will take the values of the first, second, and third elements of the returned array. You might be thinking, what if the data we destructure is less or more than the variables names we provided in the square brackets? Let’s look at those scenarios.
Destructuring with more items in the array:
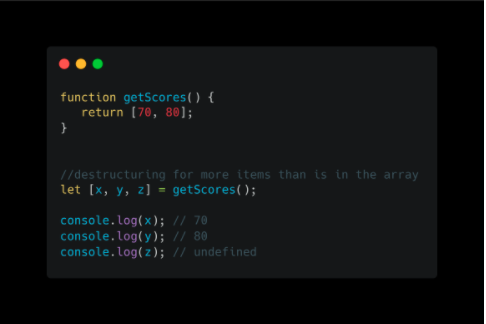
Destructuring for fewer items in the array:
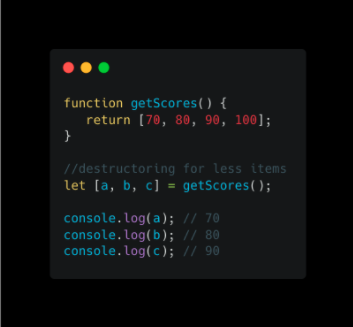
Handling ambiguity for the items in the array:
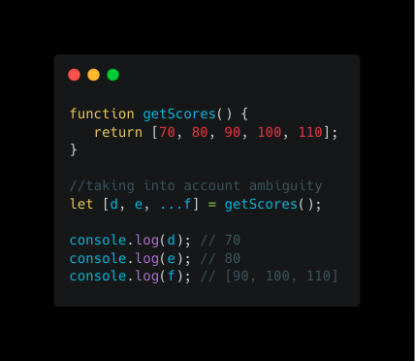
This brings us to the end of this article. I encourage you to practice these functions in a JavaScript playground like JSFiddle. See what results you get. Happy Coding 🙂